Python ord() Function with Examples | Initial Commit
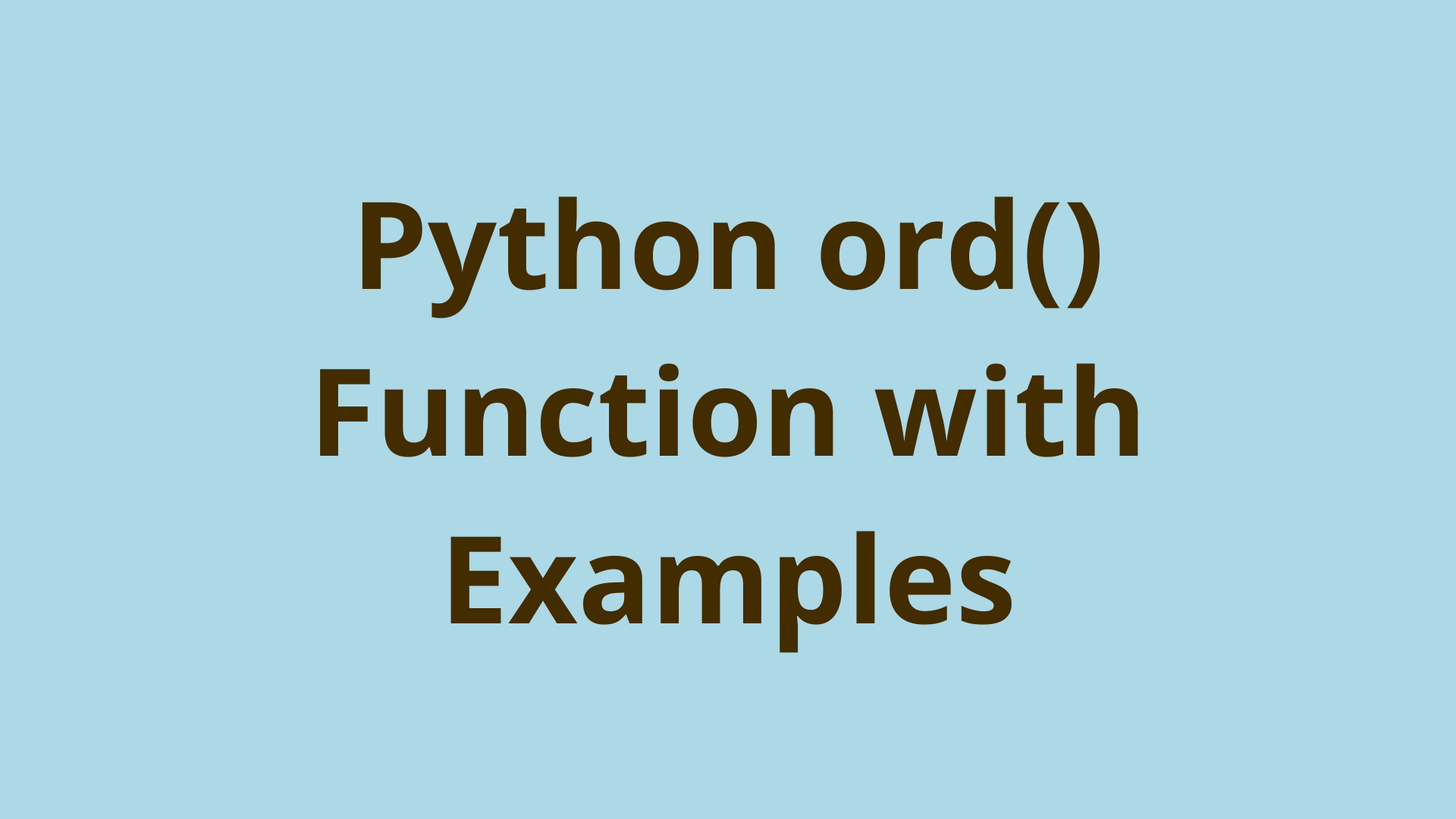
ADVERTISEMENT
Table of Contents
- Introduction
- What is ord() in Python?
- How do you use ord for a string in Python?
- How do I get ord value in Python?
- How do you get a character from ord() in Python?
- Use cases for the ord() function
- Removing specific characters from strings
- Limitations of ord()
- Last thoughts on Python ord()
- Summary
- Next Steps
- References
Introduction
An ordinal number in programming, is a one-to-one value correspondence with positive integers. Characters can be considered ordinal because we would call the character "A" the first character, "B" the second character, and so on.
In this article, you'll take an advanced look at ordinal characters in Python. More specifically, you'll learn how to apply the use of ordinal characters for string comparison. Let's get started!
What is ord() in Python?
The Python ord() function returns the Unicode value from a given character, this Unicode value is represented as an integer. This particular Python function only accepts a string with the length of one, it then returns the Unicode integer (decimal) value that represents the given string. We will go over the syntax of ord(), different use cases, and how to convert it back to its original string value.
According to Unicode, Inc. (2019), "*The Unicode Standard* is the universal character encoding standard used for representation of text for computer processing"
ASCII is a subset of *Unicode* and consists of 128 symbols in the character set. These symbols include letters (upper & lowercase), numbers, punctuation marks, special characters, and control characters
How do you use ord for a string in Python?
Compared to some built-in Python functions, the syntax of the ord() function is fairly straightforward and easy-to-use. To use the ord()
function you simply need to pass it a single string of any character, such as: ord('$')
.
Keep in mind that you will get a different numerical value for upper and lower case
How do I get ord value in Python?
Here is a simple example:
>>> ord('z')
>>> 122
As you can see, the ord()
function returns the numerical value of 122 for the lower case 'z'. If you want, you can compare this output to an ASCII chart.
Lets try using ord on an entire sentence.
>>> mySentence = "Python ord() is great!"
>>> for character in mySentence:
... print(ord(character))
...
80
121
116
104
...
You can also append these to a list to access later.
>>> listOrd = []
>>> for character in mySentence:
... listOrd.append(ord(character))
...
>>> listOrd
[80, 121, 116, 104, 111, 110, 32, 111, 114, 100, 40, 41, 32, 105, 115, 32, 103, 114, 101, 97, 116, 33]
>>>
>>> listOrd[0] == ord(mySentence[0]):
True
You could iterate through the entire list to compare values or even rebuild the entire sentence!
Comparing an integer value instead of comparing to a string value, is much easier and could potentially reduce the amount of errors your code produces
How do you get a character from ord() in Python?
To convert the integer return value of the ord()
function back to a string value, you can simply use Python's built-in chr()
function. Instead of taking a string value, it takes an integer value and returns a string value.
>>> listChr = []
>>> for item in listOrd:
... listChr.append(chr(item))
...
>>> "".join(listChr)
'Python ord() is great!'
>>>
Use cases for the ord() function
Now let's discuss some additional use cases for the ord function.
Comparing characters
As you can tell from the above examples, the ord()
function is incredibly useful for comparing characters. There is no need to mess around with clunky string indexing to compare individual characters.
>>> char1 = "a"
>>> char2 = "A"
>>> ord(char1) == ord(char2)
False
Finding permutations
Since ord() makes comparing characters and strings easier, it also makes finding permutations easier by just keeping track of the integer values instead of the string character. For example, if you wanted to find every permutation of the string "ABC".
>>> myString = "ABC"
>>> listOrd = []
>>> for character in myString :
... listOrd.append(ord(character))
>>> listOrd
[65, 66, 67]
Using this list, we can generate all of the permutations using the numeric values and then convert it back into strings to get the string permutations.
>>> permutationNums = [
(65, 66, 67),
(65, 67, 66),
(66, 65, 67),
(66, 67, 65),
(67, 65, 66),
(67, 66, 65) ]
>>> permutations = []
>>> for tup in permutationNums :
... perm = ""
... for num in tup:
... perm += chr(num)
permutations.append(perm)
>>> permutations
['ABC', 'ACB', 'BAC', 'BCA', 'CAB', 'CBA']
Removing specific characters from strings
Say you have some string where you want to remove all characters that are not lowercase alphabetical. We can loop through the string and use the ord() value to check if the character is lowercase and alphabetical. Since all of the ascii values for lowercase alphabetical characters are between 97 and 122, we will use these numbers to compare.
>>> myString = "HHhel123lo woMKrl#d"
>>> myNewString = ""
>>> for character in myString:
... asciiVal = ord(character)
... if asciiVal >= 97 and asciiVal <= 122:
... myNewString += character
>>> myNewString
"helloworld"
Limitations of ord()
Since ord()
is a simple function, it has syntax limitations. If the length of the string is more than 1, it will throw a TypeError
exception:
>>> ord('bb')
TypeError: ord() expected a character, but string of length 2 found
If you give ord an integer, it will also throw a TypeError
exception as seen below:
>>> ord(1)
TypeError: ord() expected string of length 1, but int found
As a side note, you could however make a function def paragraphToOrd(myParagraph) -> list
that ingests a whole sentence or paragraph per se and it could return a list of the Unicode values that ord() returns.
Last thoughts on Python ord()
Python's built-in ord() function can be a useful tool for the average Pythoner, or as an expert. Instead of taking the time to look up ASCII values, you can simply just use the ord()
function directly. Instead of trying to compare characters as string values, you can easily compare them as integer values. If you want to compare an entire sentence, just add the ord()
values into separate lists and compare the lists, it's that easy! Hopefully this article has pointed out some useful features of Python’s built-in ord()
function for you to use.
Summary
In this article we took a quick look at how to use the ord()
function in python. We learned the syntax of ord()
and saw how it can be used with the chr()
function to turn a sentence into its ascii values, and then back to the original string. We also took a look at some use cases for ord in python. Finally, we looked at one of the limitations of the ord function and what kind of error it throws.
You can read about the ord()
function in Python's official documentation. The ord function is part of the Python standard library. This is a collection of functions and methods that come built-in with your Python installation and are ready for you to use.
Next Steps
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
References
- Unicode Inc, The Unicode Standard: A Technical Introduction, https://www.unicode.org/standard/principles.html
- Jakub Przywóski, Python Reference (The Right Way), https://python-reference.readthedocs.io/en/latest/docs/str/ASCII.html
- Wikipedia, Unicode, https://en.wikipedia.org/wiki/Unicode
- Python Software Foundation, Built-in Functions, https://docs.python.org/3/library/functions.html#ord
Final Notes
Recommended product: Coding Essentials Guidebook for Developers